
Insight

Insight

Insight
Implementation of push notifications and in-app messaging with OneSignal
Implementation of push notifications and in-app messaging with OneSignal
Aug 26, 2024
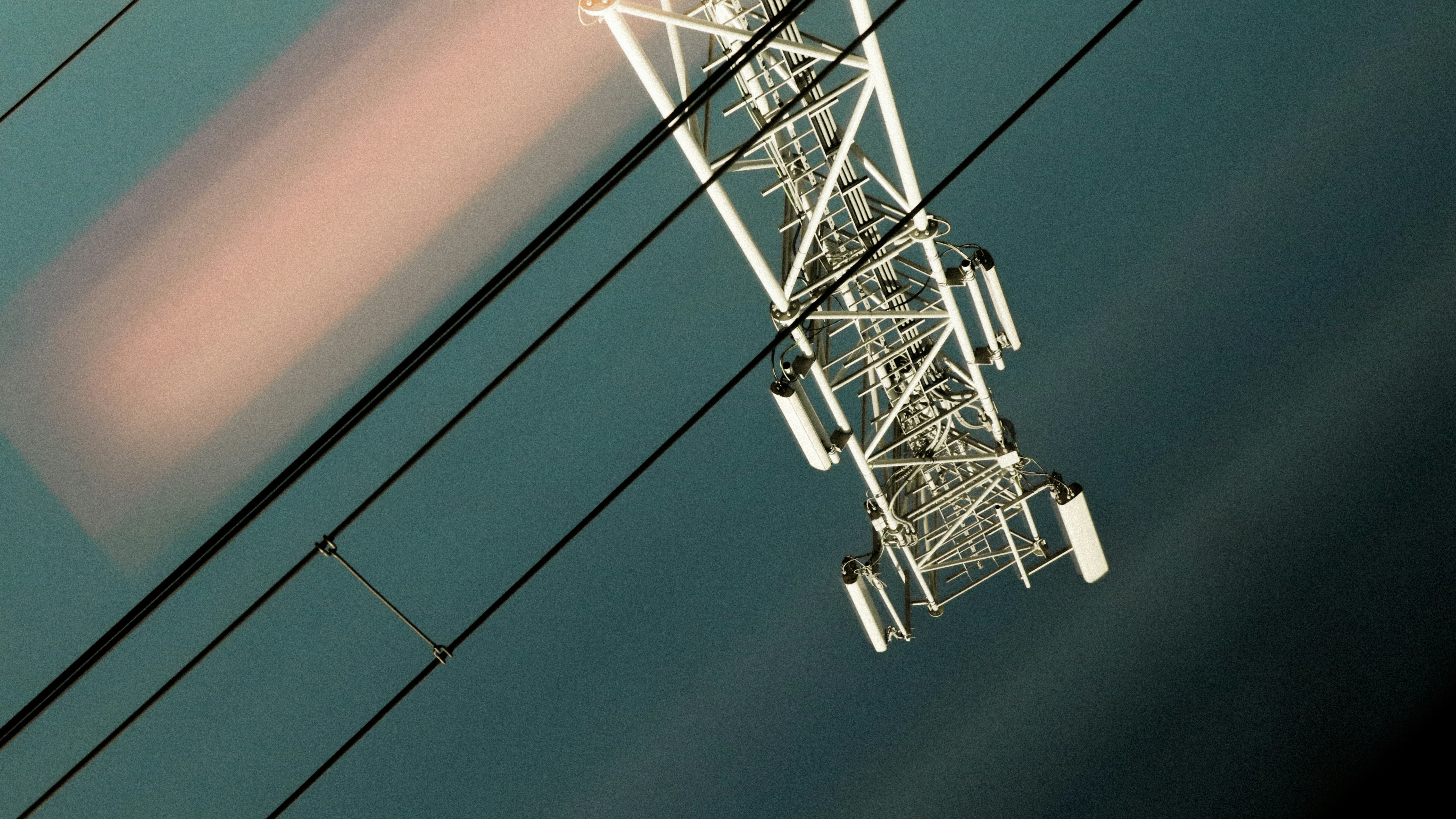
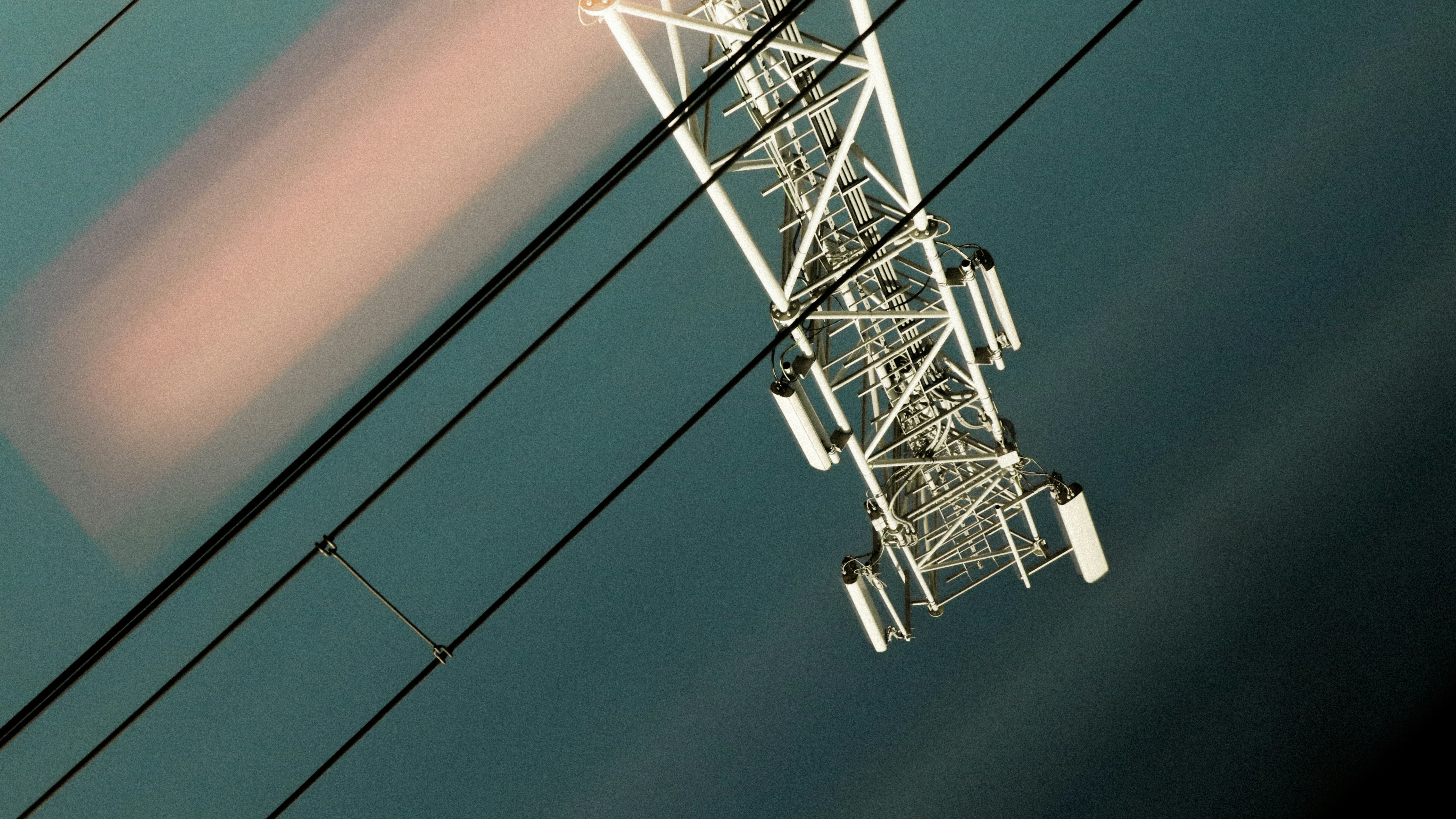
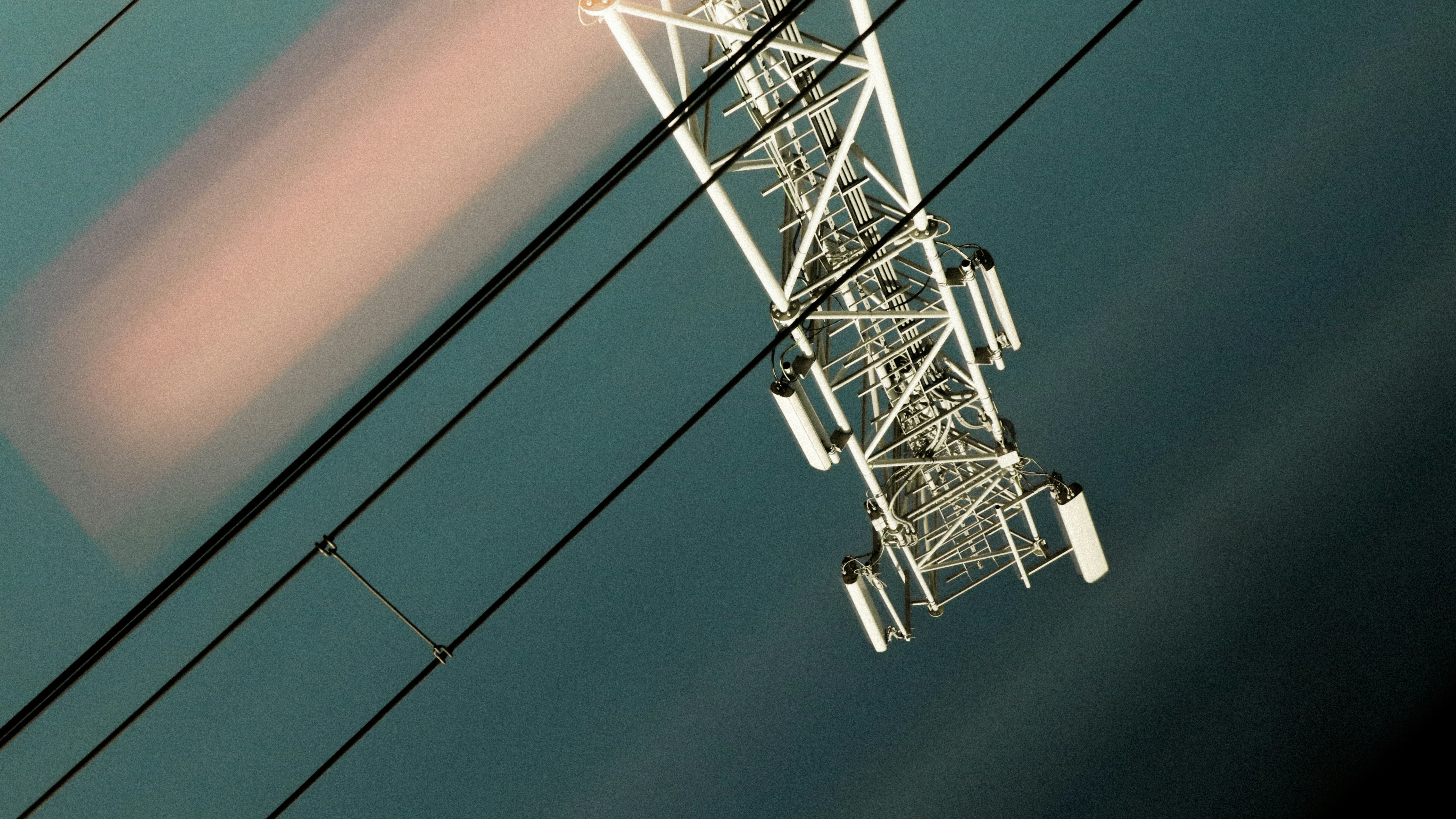
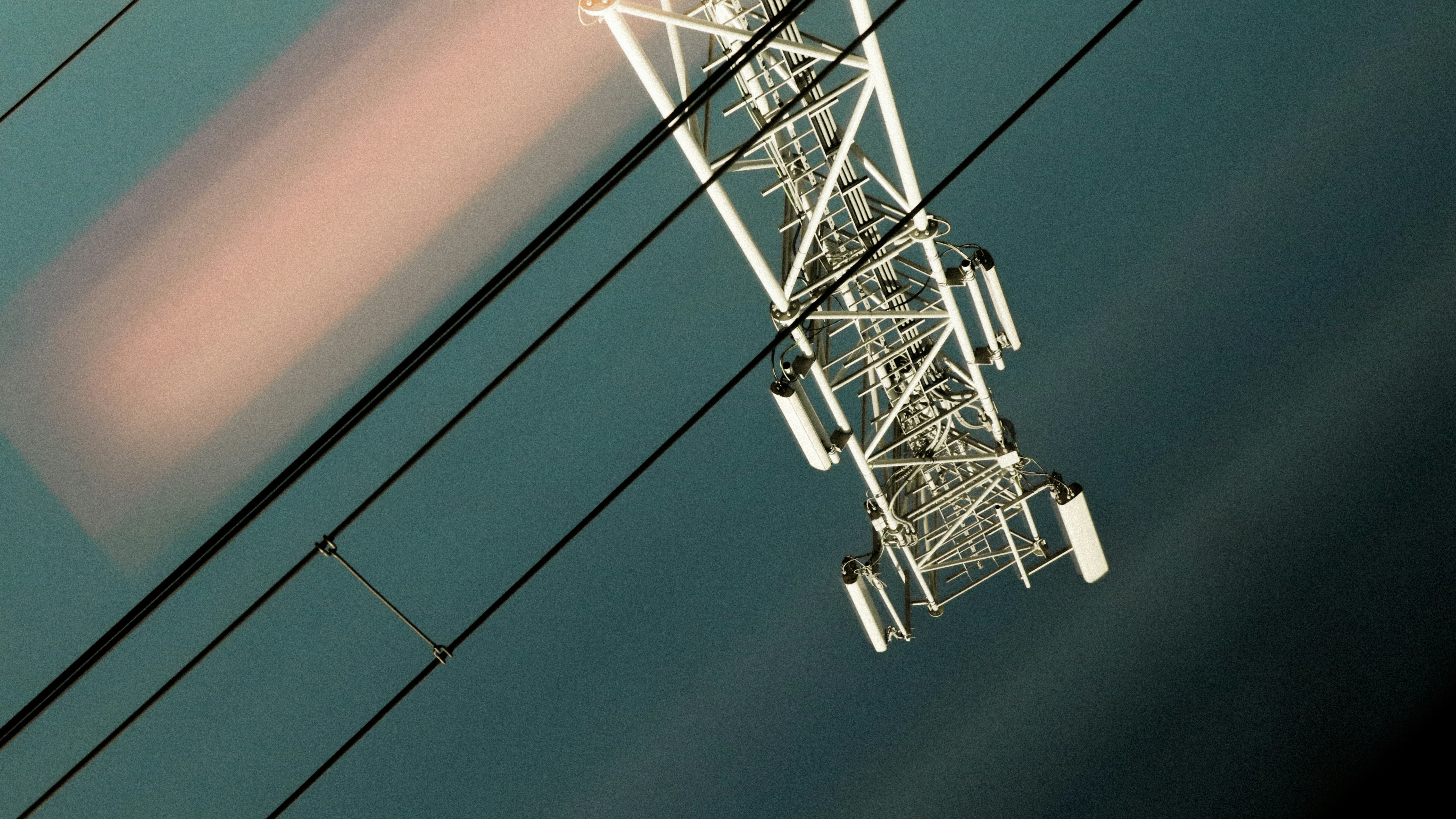
Push notifications and in-app messaging are now among the most effective tools for increasing user engagement and retention in an app. They allow you to communicate directly with users, share important information, and guide users' attention to specific content or actions. A well-implemented notification strategy can make the difference between a successful app and a neglected one.
OneSignal has established itself as one of the leading platforms for managing push notifications and in-app messaging. It offers a comprehensive solution that is easy to integrate while providing powerful features. The platform supports various operating systems, making it ideal for cross-platform Flutter apps. Additionally, OneSignal offers detailed analytics that allow you to measure the effectiveness of your messages and continuously improve them.
In this article, you will learn step by step how to integrate OneSignal into your Flutter project, set up push notifications, and implement in-app messaging. By the end of this article, you will be able to reach your users with targeted messages and significantly increase interaction with your app.
Setting Up and Configuring OneSignal
Integrating OneSignal into your Flutter project requires some basic steps to ensure that you can use push notifications and in-app messaging seamlessly. In this chapter, you will learn how to create a OneSignal account, integrate the platform into your project, and make the necessary configurations for Android and iOS.
Creating a OneSignal Account and Integrating it into the Flutter Project
The first step is to create an account with OneSignal if you don’t have one already. Visit the OneSignal website and sign up for free. After logging in, you can create a new app within the dashboard that is responsible for managing your push notifications and in-app messages.
In the next step, you need to include the Flutter SDK for OneSignal in your project. To do this, add the required dependencies in your pubspec.yaml
file:
dependencies:
flutter:
sdk: flutter
onesignal_flutter: ^5.1.2
Don't forget to update the dependencies by running the command flutter pub get
.
Cross-Platform Configuration (iOS and Android)
Android Configuration
To configure OneSignal in your Android project, you must ensure that you add the required permissions in the AndroidManifest.xml
file. This includes Internet access, receiving boot events, and permission to send push notifications.
Add the following permissions to the AndroidManifest.xml
:
<uses-permission android:name="android.permission.RECEIVE_BOOT_COMPLETED"/>
<uses-permission android:name="android.permission.INTERNET"/>
<uses-permission android:name="android.permission.WAKE_LOCK"/>
<uses-permission android:name="com.google.android.c2dm.permission.RECEIVE"
iOS Configuration
For iOS, you need to ensure that the necessary dependencies are integrated into the Podfile
. Also, make sure to configure the permissions for notifications in the Info.plist
file:
<key>UIBackgroundModes</key>
<array>
<string>remote-notification</string>
</array>
Setting Up App Keys and API Integration
To successfully integrate OneSignal, you need your specific OneSignal App Key, which you can find in the dashboard under the “App Settings” menu. This key is used in the SDK initialization to ensure that notifications are sent to the correct app.
Additionally, you can make further API integrations as needed to utilize advanced OneSignal features, such as custom segmentation or automated messages. This API integration typically occurs server-side, allowing you to communicate with your server to trigger specific events that then send a message via OneSignal.
The steps described above provide a rough overview of setting up OneSignal. For a detailed description, it's best to check the OneSignal Flutter SDK Setup. Your Flutter app is now successfully connected to OneSignal, and you are ready to implement push notifications and in-app messaging.
Implementing Push Notifications
Once the basic setup and configuration of OneSignal in your Flutter project is complete, it’s time to implement push notifications. In this chapter, you will learn how to configure the notification settings in OneSignal, handle push notifications in your Flutter project, and ultimately illustrate the entire process with sample code.
Configuring the Notification Settings in OneSignal
In the OneSignal dashboard, you can set specific notification settings for your app. Here, you configure how and when notifications should be sent to users. For example, you can specify whether messages should be sent immediately, delayed, or based on certain conditions (like reaching a goal or triggering an event).
One important feature is to segment your users. With OneSignal, you can define specific user groups that receive messages based on their behavior, preferences, or other attributes. This allows you to create personalized notifications that achieve a higher interaction rate.
Handling Push Notifications in the Flutter Project
To handle push notifications in your Flutter project, you need to ensure that the app can respond to incoming notifications even if it is closed or in the background. This requires implementing special methods that respond to notifications.
Here is a basic example of how you can handle push notifications with OneSignal in Flutter:
import 'package:flutter/material.dart';
import 'package:onesignal_flutter/onesignal_flutter.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatefulWidget {
@override
_MyAppState createState() => _MyAppState();
}
class _MyAppState extends State<MyApp> {
@override
void initState() {
super.initState();
OneSignal.initialize(Env.oneSignalAppKey);
OneSignal.Notifications.requestPermission(false);
OneSignal.login(userId)
}
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(
title: Text('OneSignal Push Notifications'),
),
body: Center(
child: Text('Warten auf Benachrichtigungen...'),
),
),
);
}
}
In this example, OneSignal is configured during the initialization of the app, permission to send push notifications is requested, and the user is logged into OneSignal.
With these few steps, you are already able to implement push notifications in your Flutter app and target your users effectively.
Implementing In-App Messaging
While push notifications are excellent for bringing users back to the app, in-app messaging allows direct communication with users while they are actively using the app. OneSignal offers a seamless way to create and manage in-app messages.
Configuring In-App Messaging in OneSignal
To use in-app messaging, you first need to ensure that this feature is activated in your OneSignal dashboard. OneSignal provides an intuitive user interface that allows you to create, design, and deliver messages to specific user segments.
Here’s how to configure in-app messaging:
Creating a New In-App Message: Navigate to the in-app messaging section in the OneSignal dashboard and create a new message. You can choose from a variety of templates or create a custom message.
Segmentation and Targeting: Similar to push notifications, you can also define specific target audiences for in-app messaging. This allows you to send messages only to users who meet certain criteria, such as users who have accessed a specific section of the app or performed a certain action.
Designing the Message: OneSignal allows you to customize the layout and design of your in-app messages. You can add images, buttons, and text to provide an engaging user experience. You can also configure the behavior of the message, such as the duration of the display or whether it has to be closed by the user.
Difference Between Push Notifications and In-App Messaging
It is important to understand that push notifications and in-app messaging cover different use cases:
Push Notifications: These are sent to the user’s device regardless of whether the app is open or not. They are ideal for encouraging users to return to the app or informing them about important events.
In-App Messaging: These messages only appear when the app is open and actively being used. They are excellent for pointing users to specific features, encouraging them to perform certain actions (e.g., trying a feature or taking advantage of an offer), or informing them about news while they are already in the app.
The interplay between both forms of communication can represent a strong strategy for increasing user engagement and retention.
Sample Code for Implementing and Using In-App Messages
Implementing in-app messaging in your Flutter project is relatively straightforward, especially if you have already integrated push notifications - it works right away!
This flexibility makes in-app messaging a powerful tool for targeting users and providing them with a personalized experience within your app.
With this implementation, you are now able to use both push notifications and in-app messaging efficiently in your Flutter app and significantly enhance user engagement.
Best Practices for Notifications and In-App Messaging
Now that you have learned the technical basics for implementing push notifications and in-app messaging in your Flutter app, it's essential to use these functions strategically and effectively. In this chapter, you will learn how to optimize the timing and relevance of your notifications, design engaging in-app messages, and continuously improve interaction rates through analysis and optimization.
Timing and Relevance of Notifications
One of the biggest challenges with push notifications is choosing the right timing and content. Here are some best practices:
Analyze User Activity: Use analytics to find out when your users are most active. Send notifications at these times to increase the likelihood that they will be opened and noticed.
Timing Coordination: Avoid sending notifications at inconvenient times, such as late at night or early in the morning. Instead, use tools like the OneSignal "Send After" feature to deliver notifications at an optimal time.
Segmentation: Use OneSignal's segmentation features to target different user groups with specific messages. This can significantly increase the relevance of your notifications, leading to better interaction rates.
Personalization: The more personal a message, the higher the chance that the user will respond. Use variables and user attributes in OneSignal to personalize content, such as by inserting the user's name or recommendations based on previous behavior.
Designing Engaging In-App Messages
In-app messages provide a direct way to communicate with your users while they are actively using the app. For these messages to be effective, they should meet the following criteria:
Visually Appealing: Ensure that your in-app messages match the app's design and are visually appealing. Use clear, eye-catching call-to-action buttons that encourage users to take a specific action.
Clear and Concise: Keep the text of in-app messages short and to the point. The user should immediately understand what is expected of them and what benefit they can gain from it.
Contextual Messages: Place in-app messages where they make the most sense. For example, if a user has not used a specific feature, you can display an in-app message encouraging them to try this feature.
Trigger-Based Messages: Use triggers to display in-app messages exactly when they are relevant to users, e.g., when they have completed a specific action or visited a certain page in the app.
Analyzing and Optimizing Engagement Rates
To maximize the success of your notification and in-app messaging strategy, continuous analysis and optimization are essential:
Tracking and Metrics: Use OneSignal dashboards to track key metrics such as open rates, click rates, and conversion rates. This data helps you understand which messages perform well and which do not.
A/B Testing: Conduct A/B tests to find out which message texts, designs, and timings resonate best with your users. Comparing different versions allows you to make informed decisions to optimize your strategy.
Collecting Feedback: Allow users to provide feedback on your messages. This can occur directly through the app or through analysis of ratings and comments in app stores.
Regular Adjustments: Remain flexible and regularly adapt your notification strategy to new insights and changes in user behavior. What works today may be outdated tomorrow; thus, continuous development is crucial.
By applying these best practices, you can ensure that your push notifications and in-app messages are perceived not only as useful but also as valuable to your users. This leads to higher user satisfaction and a long-term increase in engagement and app retention.
Conclusion
The implementation of push notifications and in-app messaging with OneSignal in your Flutter app offers an effective way to increase user engagement and enhance the user experience. By targeting users with relevant and well-timed messages, you can improve retention, foster interactions within the app, and ultimately increase the success of your app sustainably.
OneSignal represents a powerful yet easy-to-implement solution suitable for both small startups and large enterprises. From simple integration into the Flutter project to flexible configuration and analysis, OneSignal provides all the necessary tools to build a robust communication strategy.
It is essential that you consider best practices to maximize the benefits of your notifications and in-app messages. The right timing, relevance of content, and engaging design play a crucial role in this. Additionally, you should regularly analyze the performance of your messages and make adjustments to ensure optimal results.
In conclusion, push notifications and in-app messaging are no longer “nice-to-have” features but essential tools that can significantly contribute to the success of an app. With the right implementation and strategy, you can ensure that your app is not only used but also appreciated and regularly utilized.
If you need further resources or want to dive deeper into specific topics like A/B testing or advanced analyses, OneSignal offers extensive documentation and tutorials that can help you.
With this, you are well equipped to use OneSignal in your app and elevate communication with your users to the next level.
All insights
All insights
“Flutter and the related logo are trademarks of Google LLC. We are not endorsed by or affiliated with Google LLC.”
“Flutter and the related logo are trademarks of Google LLC. We are not endorsed by or affiliated with Google LLC.”
Copyright ©2025. Julian Giesen. All rights reserved.
“Flutter and the related logo are trademarks of Google LLC. We are not endorsed by or affiliated with Google LLC.”